What is the Model-View-Controller (MVC) Design Pattern
Creating well-organized, maintainable, and scalable application user interfaces is a goal for today’s interactive application developers. Applying the Model-View-Controller (MVC) design pattern is one approach to meet these objectives.
Created by Trygve Reenskaug while working as a visiting scientist at Xerox Parc Alto Research Center for Smalltalk-79, the approach introduced the concept of responsibility. MVC offers a clear and organized way to separate concerns within an application, promoting modularity and ease of maintenance.
What is the MVC Design Pattern?
The Model-View-Controller design pattern is a way to structure software applications by breaking them down into three interconnected components: Model, View, and Controller. Each component has a distinct responsibility, which helps in managing complexity and promoting the separation of concerns.
- Model: The Model represents the core data and business logic of the application. It encapsulates the data, performs operations on it, and responds to queries from the Controller and updates from the View. The Model is the backbone of the design pattern, maintaining its internal state and ensuring data consistency.
- View: The View handles presenting the data from the Model to the user in a visually appealing and informative manner. It receives data from the Model and presents it through a user interface. Importantly, the View does not contain any business logic. Its sole purpose is to display information and capture user input.
- Controller: The Controller acts as an intermediary between the Model and the View. It receives user input from the View, processes it, and interacts with the Model accordingly. The Controller handles updating the Model's data and ensuring that the View displays the correct information. It also handles application logic and flow, making decisions about how the application should respond to user actions.
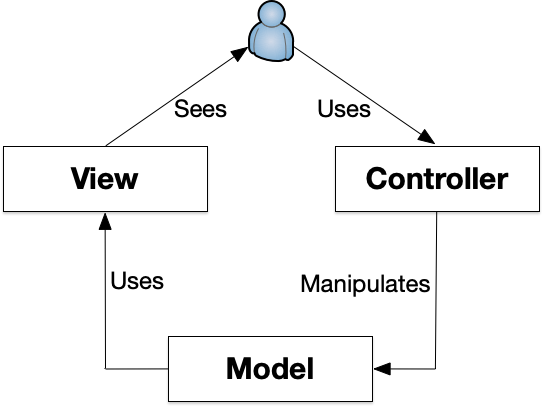
MVC Benefits
The MVC design pattern offers several benefits that contribute to the development of well-structured and maintainable applications:
- Modularity and Reusability: Each part in MVC can be developed independently and reused in other areas of the application or even in different projects. This modularity enhances code reusability and accelerates development.
- Separation of Concerns: By dividing the application into three distinct components, MVC enforces a clear separation of concerns. This approach makes it easier to manage and modify individual components without affecting the others. Developers can work on different parts of the application simultaneously, promoting collaboration and reducing conflicts.
- Improved Testability: The separation of concerns in MVC facilitates unit testing. Since the business logic remains concentrated in the Model and Controller, these components can be tested in isolation, ensuring the correctness of the application's core functionality.
- User Interface Flexibility: The View component can be easily modified or replaced without affecting the underlying business logic. This is particularly useful when adapting an application's user interface to different platforms or devices.
- Scalability: MVC's separation of concerns simplifies scaling an application. For example, if an application experiences increased user demand, the Model and Controller can be scaled independently from the View, ensuring efficient resource utilization.
Implementing MVC
Implementing the MVC pattern requires careful design and architecture considerations. Here's a simplified outline of the typical workflow within an MVC-based application:
- The user sees the information through the View, typically displaying the information on a window, browser, or other output device.
- The user interacts with the Controller, which captures user input.
- The Controller notifies with the Model if needed, and updates the Model's state.
- The Model notifies the View about changes in its data, and the View updates its display accordingly.
- The user sees the updated information in the View.

Frameworks and libraries, such as Ruby on Rails, Django, and Angular, provide built-in support for MVC, streamlining the development process and adhering to best practices for web and mobile applications.
Competing Approaches
While the Model-View-Controller (MVC) design pattern is widely used and proven effective, there are several competing strategies and alternative architectural patterns that have emerged over time. Each competing strategy addresses certain aspects of application design, and the choice between them depends on the specific requirements of the project. Here are a few competing strategies:
- Model-View-ViewModel (MVVM): MVVM is an evolution of the
MVC pattern and is particularly popular in the context of building
user interfaces for web and mobile applications. In MVVM, the
ViewModel acts as an intermediary between the Model and the View.
It exposes data and commands that the View binds to, allowing for
a more declarative approach to UI development. MVVM focuses on
data binding and often facilitates easier unit testing of the UI
logic.
Model-View-ViewModel Diagram - Model-UI-delegate: The delegate in this model tightly
couples the view and controller. The combined components, called
the UI delegates manage information about how to draw the component
on the screen. In addition, the UI delegate reacts to various
events that propagate through the component. Java Swing uses this
approach.
Model-UI-Delegate Diagram - Model-View-Presenter (MVP): MVP is another variation of
MVC that places a stronger emphasis on separating the presentation
logic from the user interface. In MVP, the Presenter acts as an
intermediary, handling user input and interacting with the Model.
Unlike MVC, the View is passive in MVP and delegates most of its
functionality to the Presenter. This pattern is often used in
scenarios where the UI needs to be more independent for testing or
when dealing with legacy code.
Model-View-Presenter Diagram - Flux Architecture: The Flux architecture, developed by
Facebook for managing state in web applications, emphasizes
unidirectional data flow. It is particularly useful for
applications with complex user interfaces and a need for
consistent state management. Flux separates the data flow into
distinct actions, dispatchers, stores, and views. Redux, a
popular library, is based on the Flux architecture and is
commonly used with React applications.
Flux Architecture Diagram - Component-Based Architecture: Component-based
architecture, often seen in frameworks like React and Angular,
structures applications around reusable components. Each component
encapsulates both its user interface and logic. This approach
promotes modularity and reusability, allowing developers to create
complex applications by composing smaller, self-contained
components.
Component-Based Diagram - Model-View-Intent (MVI): MVI is an architecture pattern
that originated in the Android development community. It is
similar to MVVM and focuses on unidirectional data flow. In MVI,
the Intent represents user actions that trigger updates in the
Model and ultimately lead to changes in the View. This pattern
aims to provide a clear separation of concerns and to simplify
UI logic.
Model-View-Intent Diagram
Each of these strategies has its strengths and weaknesses, and the choice depends on factors such as the nature of the application, the development team's familiarity with the pattern, and the desired level of separation between different components. It's worth noting that many modern frameworks and libraries provide flexibility to adapt and mix these patterns according to the specific needs of the project.
Conclusion
The Model-View-Controller design pattern remains a fundamental concept in software architecture due to its ability to promote organization, modularity, and maintainability. By separating concerns and creating well-defined roles for each component, MVC enables developers to create robust applications that are easier to understand, extend, and maintain. Whether building a simple application or a complex software system, understanding and implementing the MVC pattern can significantly contribute to a successful software development process.
Connect: